What Is ReactJS?
Starting this tutorial with assuming that you have some familiarity with HTML and JavaScript & also assume that you’re familiar with programming concepts like functions, objects, arrays, and about classes.
Now I continue with ReactJS:
- React Is Nothing but a JavaScript Library, which was created by Facebook in 2011.
- React encourages the creation of reusable UI components.
- React will efficiently update and render(display) just the right components when your data changes over time.
- React follow uni-directional data flow, which reduces the boilerplate and reasonably easier than traditional data binding.
- It powers up mobile apps using React Native.
Terms & Features of ReactJS:
1. JSX (JavaScript XML) :
- JSX is an XML/HTML like extension to JavaScript. It isn’t necessary to use this, but it is recommended in React. Exm:
const val= <h1> Hello Message ! </h1>
- As shown above, JSX syntax is neither in JavaScript nor HTML.
- Like HTML, JSX tags have a tag name, attributes & children.
- It also comes with the full features of ES6( ECMAScript 2015 ).
- If an attributes wrapped in curly braces, then that is a JavaScript expression.
2. React DOM Render :
- The method ReactDOM.render() is used to display the HTML Element’s :
<div id="root">Hello World!</div> <script type="text/babel"> ReactDOM.render( <h1>Hello React!</h1>, document.getElementById('root')); </script></pre>
3. React Components:
- React is all about the components.
- React Components are nothing but similar to the JavaScript Functions.
- The function name is known as the React Components (As shown Below “iTechinsiders” is a React Components)
Exm:
<div id="root"></div> <script type="text/babel"> function iTechinsiders() { return <h1>Hello React!</h1>; } ReactDOM.render(<iTechinsiders/>, document.getElementById('root')); </script>
4. JSX Expression ;
- Expression wrapping in Curly braces {} in JSX, known as JSX Expression.
Exm:
<div id="root">Hello World!</div> <script type="text/babel"> const name= 'itech'; ReactDOM.render( <h1>Hello {name}!</h1>, document.getElementById('root')); </script>
5. Babel:
- Babel allows using the newest feature of JavaScript(ES6).
- Babel is a JavaScript Compiler which converts markup/programming lang into JavaScript (Means Browser does not understand JSX format that’s why it needs to convert into JavaScript).
#Adding React to an HTML Page:
<!DOCTYPE html> <html lang="en"> <title>Test React</title> <!-- Load React API --> <script src= "https://unpkg.com/react@16/umd/react.production.min.js"></script> <!-- Load React DOM--> <script src= "https://unpkg.com/react-dom@16/umd/react-dom.production.min.js"></script> <!-- Load Babel Compiler --> <script src="https://unpkg.com/babel-standalone@6.15.0/babel.min.js"></script> <body> <script type="text/babel"> // JSX Babel code goes here </script> </body> </html>
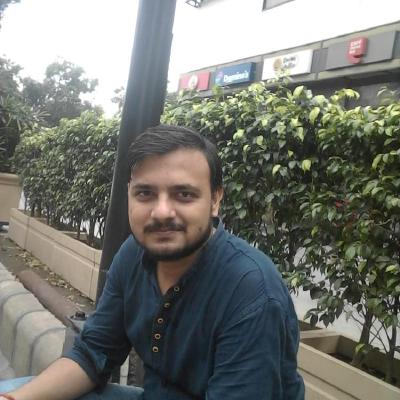
Praveen Maurya work in e-commerce domain having knowledge of Plugin development in Magento 1.x/2.x, Drupal, Woo-Commerce, PrestaShop, Opencart and other e-commerce solution. When he is not engrossed with anything related to these, he loves to explore new things.
Saved as a favorite!, I like it!