How to use geo-location plugin in react native android studio:
Here we are going to discuss the topic “How to use geo-location plugin in react native“, using geo-location plugin you find the current location of user.
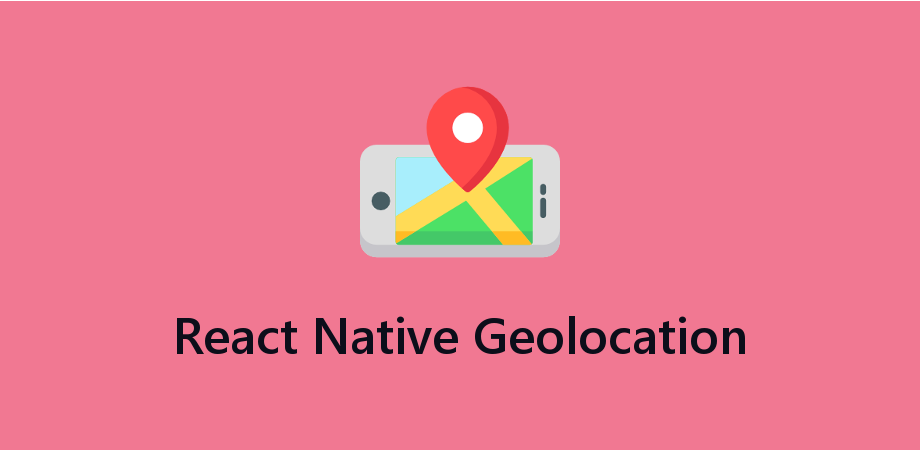
Please follow all the steps carefully, let’s start.
Step1:
Firstly we need to install the geolocation plugin.
npm install react-native-geolocation-service
Step2:
Now we need to link the plugin if your version of react native less than 0.60
react-native link react-native-geolocation-service
Step3: How to check the geolocation plugin is properly linked or not.
Because plugin linking is the most important part of app, if plugin is not linking properly then you not able to find the location of user, so check all the files carefully, let’s start.
android/app/build.gradle:
Please, find these lines if they are present in the build.gradle then no issue but if not then add these lines in dependencies.
dependencies {
implementation project(‘:react-native-geolocation-service’) }
build.gradle(project label):
check these lines in project label build.gradle if it already added then no issue otherwise you need to add this line googlePlayServicesVersion = “16.0.0”
ext {
compileSdkVersion = 28
targetSdkVersion = 28
buildToolsVersion = “28.0.3”
supportLibVersion = “28.0.0” //these all are already added
googlePlayServicesVersion = “16.0.0” //add this line only
}
android/setting.gradle:
Now you check your setting.gradle files, if already linked then no need to add otherwise add these lines
include ‘:react-native-geolocation-service’
project(‘:react-native-geolocation-service’).projectDir =
new File(rootProject.projectDir,‘../node_modules/react-native-
geolocation-service/android’)
App/Java/MainApplication.java:
Now we check the file App/Java/MainApplication.java for the import section.
import com.agontuk.RNFusedLocation.RNFusedLocationPackage;
and then check in
@overridepublic class MainApplication extends Application implements ReactApplication {
@Override protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new RNFusedLocationPackage() // check for these line if add
then fine otherwise add it
);
}
}
Now we are ready to use this plugin in our project but we first need to accept the permission, so let’s start.
Permission Checking:
App/manifests/androidmanifest.xml –
Checking these lines for granting permission.
<uses-permission android:name=“android.permission.ACCESS_FINE_LOCATION” />
Now we are ready to implement location plugin in our application, so first we open a file where we want to implement the plugin, firstly we need to add the code for asking about permission.
Implement the code –
import { PermissionsAndroid } from ‘react-native’;
import Geolocation from ‘react-native-geolocation-service’;
var hasLocationPermission = PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.ACCESS_FINE_LOCATION,{
‘title’: ‘ACCESS_FINE_LOCATION’,
‘message’: ‘This app would like to view your ACCESS_FINE_LOCATION.’
}
)
export default class CurrentLocation {
constructor() { }
gettingUserCurrentLocation() {
if (hasLocationPermission) {
Geolocation.getCurrentPosition(
(position) => {
console.log(position)
}
else{
console.log(error)
// See error code charts below.
},
{ enableHighAccuracy: true, timeout: 15000, maximumAge: 10000 }
)}}
So we completed the topic “How to use geo-location plugin in react native“.
You can find my post on medium as well click here please follow me on medium as well.
You can find my next post here.
If have any query/issue, please feel free to ask.
Happy Coding Guys.
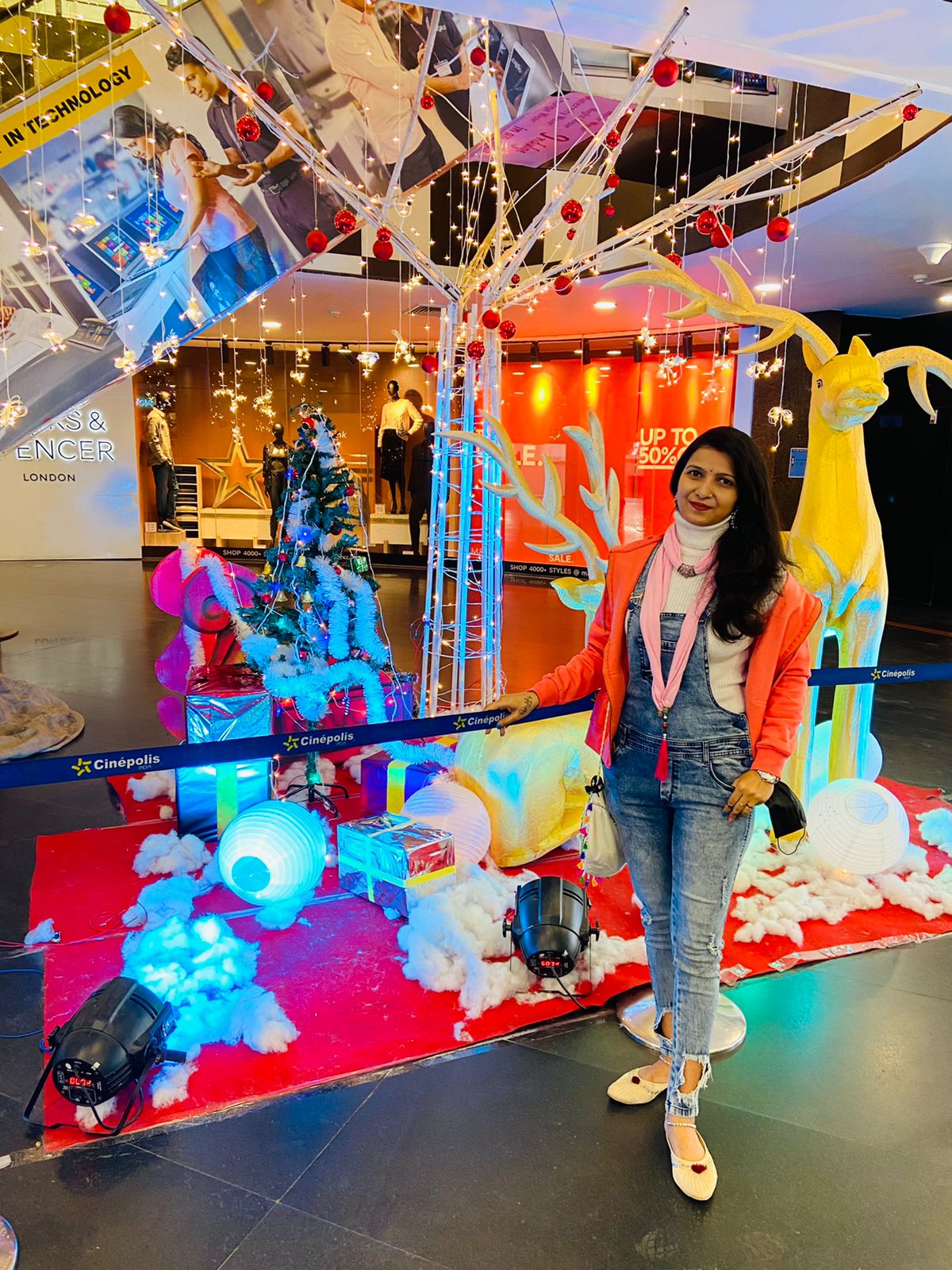
Hi, I am a professional Ionic and React Native Pixel Perfect App Designer and Developer, with expertise in Client Communication, Bug Fixing, Third Party Lib, Version Control Tools, Requirement Understanding, and managing teams, I have 6+ years of experience in the same domain as well as in Codeigniter, JS, IoT, and more than 10 other languages. For the last 6+ years, not a single day went without design/development.
Please follow me on Medium: https://nehadwivedi1004.medium.com/