Creating registration form with validators and rest API:
Here we are going to discuss the topic “Registration form using form builder, validator and rest api“, we create the registration form using rest api for storing data.
Output Example –
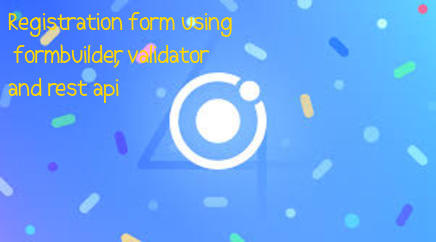
Creating any form in ionic we need following ionic components, formbuilder, formgroup, formcontrols and validators.
For calling rest api we need httpmodule, in this we use form builder, validators and slider.
Step 1:
Firstly we need to create the ionic project, ionic provide some predefined templates.
ionic start signup blank –type=ionic-angular
Step 2:
firstly we import register page and httpmodule in app.module.ts file, just go to the src/app/app.module.ts
import { HttpModule } from '@angular/http';
@NgModule({
declarations: [
MyApp,
SignupPage ,
],
bootstrap: [IonicApp],
entryComponents: [
MyApp,
SignupPage ,
],
imports: [
HttpModule,
IonicModule.forRoot(MyApp),
],
Step 3:
Now we are ready to design the register page, so goto the path where you want integrate this.
FormControl it is a value that user entered(basically name of form input field).
FormGroup is collection of FormControls.
validation – validation is a important part of any form, we use the validation for validate the input field, these are the some validation types.
- Validators.required
- Validators.minLength(number)
- Validators.maxLength(number)
- Validators.pattern(‘patternʼ)
FormBuilder – use to make the process of creating FormGroup.
Step 4:
Here we start to using formbuilder in src/pages/signup/signup.html
<ion-header>
<ion-navbar color="primary">
<ion-title>Signup</ion-title>
</ion-navbar>
</ion-header>
<ion-content padding>
<ion-list>
<ion-card>
<ion-card-content>
<form [formGroup]="formgroup" (ngSubmit)="submit(formgroup)">
<ion-item>
<ion-label fixed>Name</ion-label>
<ion-input type="text" text-left formControlName="firstname"></ion-input>
</ion-item>
<ion-item *ngIf="!formgroup.controls.firstname.valid && (formgroup.controls.firstname.dirty)">
*Name is required
</ion-item>
<ion-item>
<ion-label fixed>Email Adresse</ion-label>
<ion-input type="email" text-left formControlName="email"></ion-input>
</ion-item>
<ion-item *ngIf="!formgroup.controls.email.valid && (formgroup.controls.email.dirty )">
*Email Adresse is required
</ion-item>
<ion-item>
<ion-label fixed>Password</ion-label>
<ion-input type="password" text-left formControlName="pass"></ion-input>
</ion-item>
<ion-item *ngIf="!formgroup.controls.pass.valid && (formgroup.controls.pass.dirty)">
*password is required
</ion-item>
<button ion-button full color="primary">Register Now</button>
</form>
</ion-card-content>
</ion-card>
</ion-list>
</ion-content>
Step 5:
Now we generate .ts file and see how to submit formdata using rest api, now goto the path src/pages/signup/signup.ts
import {Component} from '@angular/core';
import {IonicPage, NavController, NavParams, Platform} from 'ionic-angular';
import {Http} from '@angular/http';
import 'rxjs/add/operator/catch';
import 'rxjs/add/operator/toPromise';
import {Validators, FormBuilder, FormGroup} from '@angular/forms';
@IonicPage()
@Component({
selector: 'page-signup',
templateUrl: 'signup.html',
})
export class SignupPage {
formgroup: FormGroup;
firstname: any;
email: any;
fatchdata: any = [];
password: any;
constructor(public navCtrl: NavController, public formbuilder: FormBuilder) {
this.formgroup = formbuilder.group({
firstname: ['', Validators.required],
pass: ['', Validators.required],
email: ['', Validators.required],
});
}
submit(formgroup) {
var plink = 'https://domain/api/register';
var data_l = {name: formgroup.value.firstname,
email: formgroup.value.email,
pass: formgroup.value.phone,
}
this.http.post(plink,data_l ).map(res=>res.json())
.subscribe(data=> {
this.fatchdata = data;
if (this.fatchdata['status'] == '200') {
let alert = this.alertCtrl.create({
title: 'Success Massage',
message: this.fatchdata['msg'],
buttons: [{
text: 'Ok',
role: 'ok',
handler: () => {}
}]
});
alert.present();
}
else {
let alert = this.alertCtrl.create({
title: 'Unsuccess Massage',
message: this.fatchdata['msg'],
buttons: [{
text: 'Ok',
role: 'ok',
handler: () => {
}
}]
});
alert.present();
}
}, error => {
});
}
Step 6:
Now we are going to add the platform as well as create the APK.
ionic cordova resources android –splash
ionic cordova resources android –icon
ionic cordova platform add android
ionic cordova build android
Step 6:
Now APK is ready to use and you can test your app with registration process as well as API.
Why i choose ionic –
I’m a big fan of ionic with angular, because it is very easy to implement complicated concepts and designs, in above example i am explain you in detail how to create a very simple registration form.
So we complete the tutorial which is “Registration form using form builder, validator and rest api“.
You can find my post on medium click here please follow me on medium as well.
You can find my next post here.
If have any query/issue, please feel free to ask.
Happy Coding Guys.
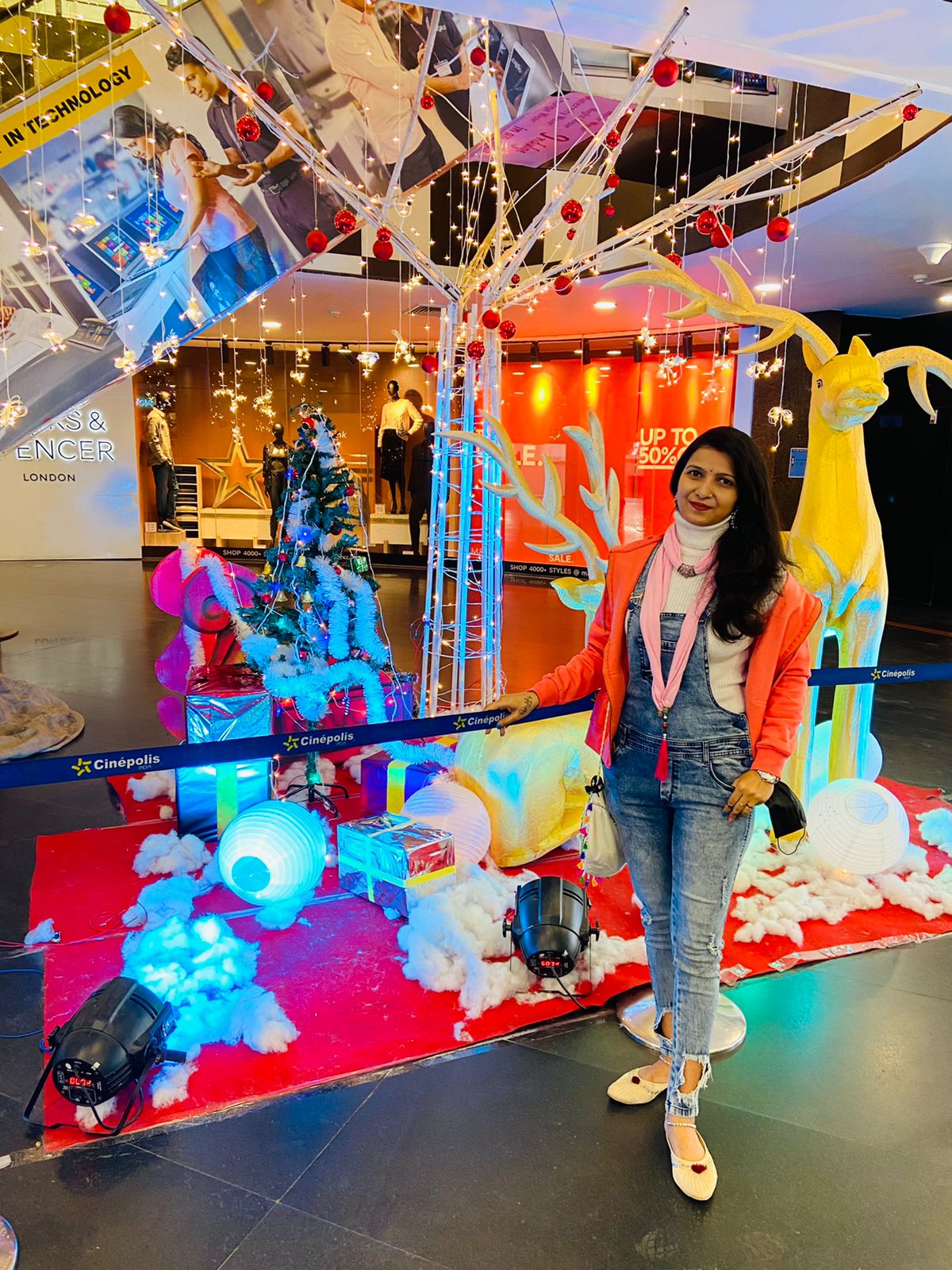
Hi, I am a professional Ionic and React Native Pixel Perfect App Designer and Developer, with expertise in Client Communication, Bug Fixing, Third Party Lib, Version Control Tools, Requirement Understanding, and managing teams, I have 6+ years of experience in the same domain as well as in Codeigniter, JS, IoT, and more than 10 other languages. For the last 6+ years, not a single day went without design/development.
Please follow me on Medium: https://nehadwivedi1004.medium.com/